In embedded RTOS operating systems, real-time tasks are typically implemented as infinite loops.
void task_code(void *pdata)
{
while (True) {
… task code …
task_suspend(task_period);
}
}
In this model, each loop cycle involves the task executing its code and then being suspended for the duration of the task’s period. For example, in an embedded RTOS operating system, a task responsible for reading sensor data could have its code execution within the infinite loop. This task would continuously read data from the sensors, process it, and then suspend itself until the next cycle.
Using this task structure, the task code is not executed periodically but rather at a constant interval between the completion of one iteration and the beginning of the next. RTOSs call this suspension interval the task period, but this term does not strictly correspond to the definition in real-time terminology.
In real-time terminology, the concept of the period of a real-time task is closely related to the sampling period of Whittaker–Nyquist–Shannon‘s theorem. This theorem asserts that the information of a physical signal can be retained through periodic sampling, laying down the conditions of periodicity necessary for this preservation. Real-time tasks executed with strict periods can properly preserve and process the information of the physical signals.
If the time interval between successive executions of a task depends on its own execution time, and also on the execution time of higher priority tasks that need to be executed, then the conditions of Whittaker-Nyquist-Shannon’s theorem for preserving information from the physical domain are not guaranteed. Real-time tasks implemented as infinite loops do not execute periodically (they are only suspended for a time known as the period) and therefore put at risk the fulfillment of the conditions of Whittaker-Nyquist-Shannon’s theorem.
Periodic real-time task in GeMRTOS #
GeMRTOS supports tasks implemented as infinite loops and also offers the definition of real-time tasks with periodic invocations. These real-time tasks are released at regular intervals, maintaining a consistent time gap between consecutive invocations as specified by the task period. This feature allows for the implementation of physical signal processing tasks, ensuring data integrity in accordance with the Whittaker-Nyquist-Shannon theorem.
The code for a periodic real-time task in GeMRTOS consists of a straightforward function that GeMRTOS will release at regular intervals (by setting the period of the task):
void task_code(void *pdata)
{
… task code …
}
A periodic task may experience jitter due to the execution of higher-priority tasks, but it eliminates the drift that is unavoidable in infinite loop tasks.
Processing of physical world signals #
Real-time systems engage with their surroundings, involving interaction with physical signals that need to be sampled according to the sampling theorem to retain all the information from the environment.
To apply the sampling theorem effectively, it is useful to view physical signals as combinations of sinusoidal functions, made possible by the mathematical principles of the Fourier series and transform, which assert that any function can be represented as a combination of sine and cosine waves.
The Fourier series states that a continuous periodic signal can be expressed as:
\(F(t)=\frac{a_0}{2} +\sum_{n=1}^{\infty} [a_n\cdot \cos(\frac{2\cdot \pi \cdot n}{T} \cdot t) + b_n \cdot \sin(\frac{2\cdot \pi \cdot n}{T} \cdot t)] \)
where
– \( a_0 \) is the constant coefficient,
– \( a_n \) and \( b_n \) are the coefficients of the cosine and sine terms (the Fourier coefficients), respectively,
– \( T \) is the period of the input signal,
– \( n \) is the frequency index, and
– \( t \) is the time.
All real-world signals do not require an infinity of frequencies. While some signals may require a wide range of frequencies to accurately represent them, always this range is finite for real-world signals. As a result, real-world signals can be expressed by limiting the frequency index (n) to a finite value, for instance N. This maximum frequency index N will determine the bandwidth of the periodic signal and, consequently, the maximum frequency needed to express the signal using the Fourier series (and similarly with the Fourier transform).
NOTE: Because frequency response analysis offers important insights into how a system reacts to various frequencies of input signals, it is widely employed in signal processing. Engineers can learn how a system amplifies or attenuates signals at various frequencies by examining its frequency response. To obtain desired performance characteristics, this information is essential for building filters, equalizers, and other signal processing components. Frequency response analysis is a crucial tool for signal processing applications because it enables engineers to determine resonant frequencies, stability problems, and other significant system aspects.
With this in mind, Whittaker–Nyquist–Shannon‘s theorem states that a real-world signal (belonging to the continuous-time domain) can be sampled if it is sampled at more than twice the maximum frequency of the signal (the frequency with the N-index). The samples produced by the Whittaker–Nyquist–Shannon‘s theorem preserve all the information of the signal from the continuous-time domain to the discrete-time domain, where the information can be processed by the real-time system.
The figure below shows the various fundamental aspects involved in processing real-world signals within a real-time system.
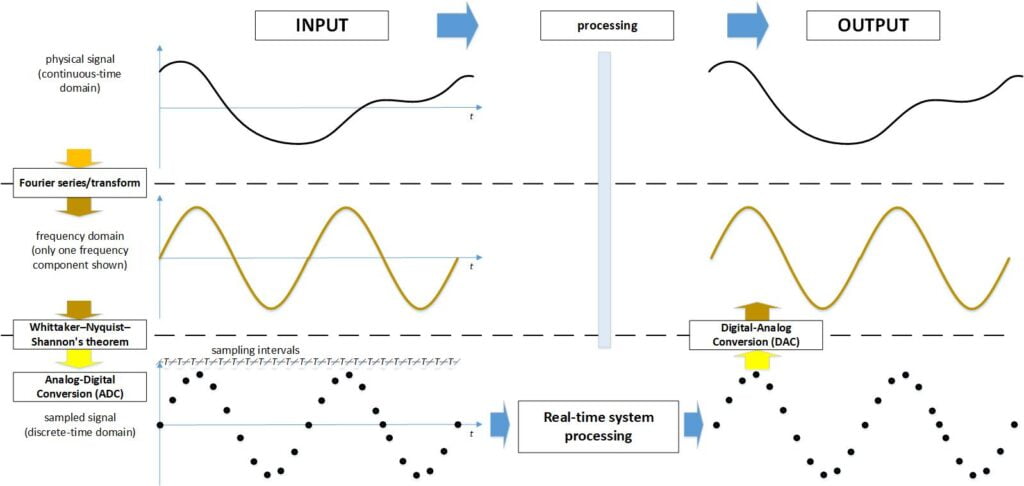
For simplicity, the real-time processing depicted in the figure merely involves copying the input value to the output value, resulting in an output signal that is identical to the input signal.
It’s evident that when the sampling interval fails to meet the conditions of the Whittaker–Nyquist–Shannon theorem, disturbances will be introduced, impacting the output signal. Inherently, real-time tasks executed as infinite loops do not adhere to the conditions of the Whittaker–Nyquist–Shannon theorem.
To illustrate the concept of disturbances caused by infinite-loop tasks, let’s focus on generating an output sinusoidal signal from a predefined table. This table comprises N values of the sinusoidal signal, sampled at regular intervals. Each time the task is called, the output is updated with the next value from the table. Once the task exhausts the table, it loops back to the first value and continues the process indefinitely. If the task were executed periodically, with a period equal to T, then the sinusoidal signal would have a period equal to:
\(Period_{sinusoidal \space signal} = N \cdot T \)However, if we consider an infinite-loop task and set the suspended time equal to T, the period of the sinusoidal output would also depend on the execution time of each invocation of the task:
\(Period_{sinusoidal \space signal} = N \cdot T + \sum_{i=1}^{N} C_i\)
where:
– \( C_i \) is the execution time of the invocation \( i \) of the task.
Hence, the continuous execution of the infinite-loop task introduces frequency perturbations in the output signal. These perturbations vary based on the task’s execution time, potentially causing deviations from the expected frequency response of the system.
Conclusions #
Most embedded RTOSs implement real-time tasks as infinite-loop functions suspended for a time interval equal to their period. This approach offers advantages from a real-time perspective by balancing system load: each task allocates time equal to its period for other tasks, ensuring fair execution regardless of individual task execution times. This approach enhances system efficiency and responsiveness by preventing any single task from monopolizing system resources. Thus, it promotes smooth and predictable operation in real-time applications.
From a frequency analysis perspective, infinite-loop tasks can introduce perturbations that potentially impact the dynamics of the physical applications associated with the real-time system. These perturbations represent additional actions transferred to the application, not initially accounted for during system design. Ultimately, they manifest as energy transferred to the application, which can lead to undesirable behaviors such as vibration, noise, heat generation, electromagnetic interference.
On the other hand, periodic real-time tasks enhance efficiency from a frequency analysis standpoint. However, thorough worst-case load analysis is necessary to assess the schedulability of the real-time system. It’s important to note that the suitability of each approach—whether infinite-loop or periodic tasks—depends on the specific requirements and perspectives involved. GeMRTOS supports both alternatives, enabling them to run concurrently within the same scheduling list or in separate ones as needed.