Introduction #
The concept of a "trigger resource" serves as a generalization of interrupts, enabling tasks to be resumed or restarted when a specific event occurs. Tasks can be registered with a trigger resource, and when the trigger resource is activated, the associated tasks are resumed or restarted. The gu_TriggerCreate function creates a trigger resource and allows associating an external interrupt with it. The gu_TriggerRegisterTask function registers a task with a trigger resource. When the trigger resource is activated, it is disabled until all registered tasks that have been resumed or restarted transition to a waiting state for a new trigger. Tasks associated with a trigger resource enter a waiting state for the trigger when they finish execution. Additionally, the gu_TriggerWait function explicitly places a task into the waiting for the trigger state. Trigger resources can be associated with external events, such as hardware interrupts. In this case, all tasks registered with the trigger resource behave as Interrupt Service Routines (ISRs). Importantly, these tasks operate independently of each other, ensuring that multiple tasks can be associated with a single trigger resource. Trigger resources can also be triggered from tasks' code using the gu_TriggerRelease function. When triggered, all registered tasks will be resumed or restarted if the trigger resource is enabled. The gu_TriggerEnable function enables a trigger resource, while the gu_TriggerDisable function disables it.
Enable and disable hook functions #
The system provides flexibility in handling events by allowing hook functions to be executed when trigger resources are enabled or disabled. The gu_TriggerEnableHook function allows configuring a function to be executed before a trigger resource is enabled, while gu_TriggerDisableHook configures a function to be executed after the trigger resource is disabled. These hook functions are intended to execute specific code associated with the devices associated with the trigger resource.
Trigger flexibility #
The system provides flexibility in handling events by allowing hook functions to be executed when trigger resources are enabled or disabled. The gu_TriggerEnableHook function allows configuring a function to be executed before a trigger resource is enabled, while gu_TriggerDisableHook configures a function to be executed after the trigger resource is disabled. These hook functions are intended to execute specific code associated with the devices associated with the trigger resource. Trigger resources offer a flexible approach to handling system events, overcoming the limitations of traditional interrupts. For example, Universal Asynchronous Receiver/Transmitter (UART) devices, like many peripherals, typically group various internal events, such as input buffer full and output buffer empty, into a single hardware interrupt signal. This requires the UART driver to determine which event triggered the interrupt and execute the corresponding code. With trigger resources, a trigger resource can be associated with the UART interrupt signal. The task code registered to this trigger resource then checks which event triggered the interrupt: input buffer full, output buffer empty, or both. If the input buffer is full, the trigger resource is activated, resuming all tasks associated with reading from the buffer. This example demonstrates the flexibility of trigger resources by allowing for the specific handling of individual events within a single interrupt signal. This approach eliminates the need for complex logic within the interrupt service routine to determine the cause of the interrupt and execute the appropriate actions. By leveraging trigger resources and hook functions, the GeMRTOS system provides a powerful and flexible mechanism for managing system events, enhancing the efficiency and modularity of the overall system design.
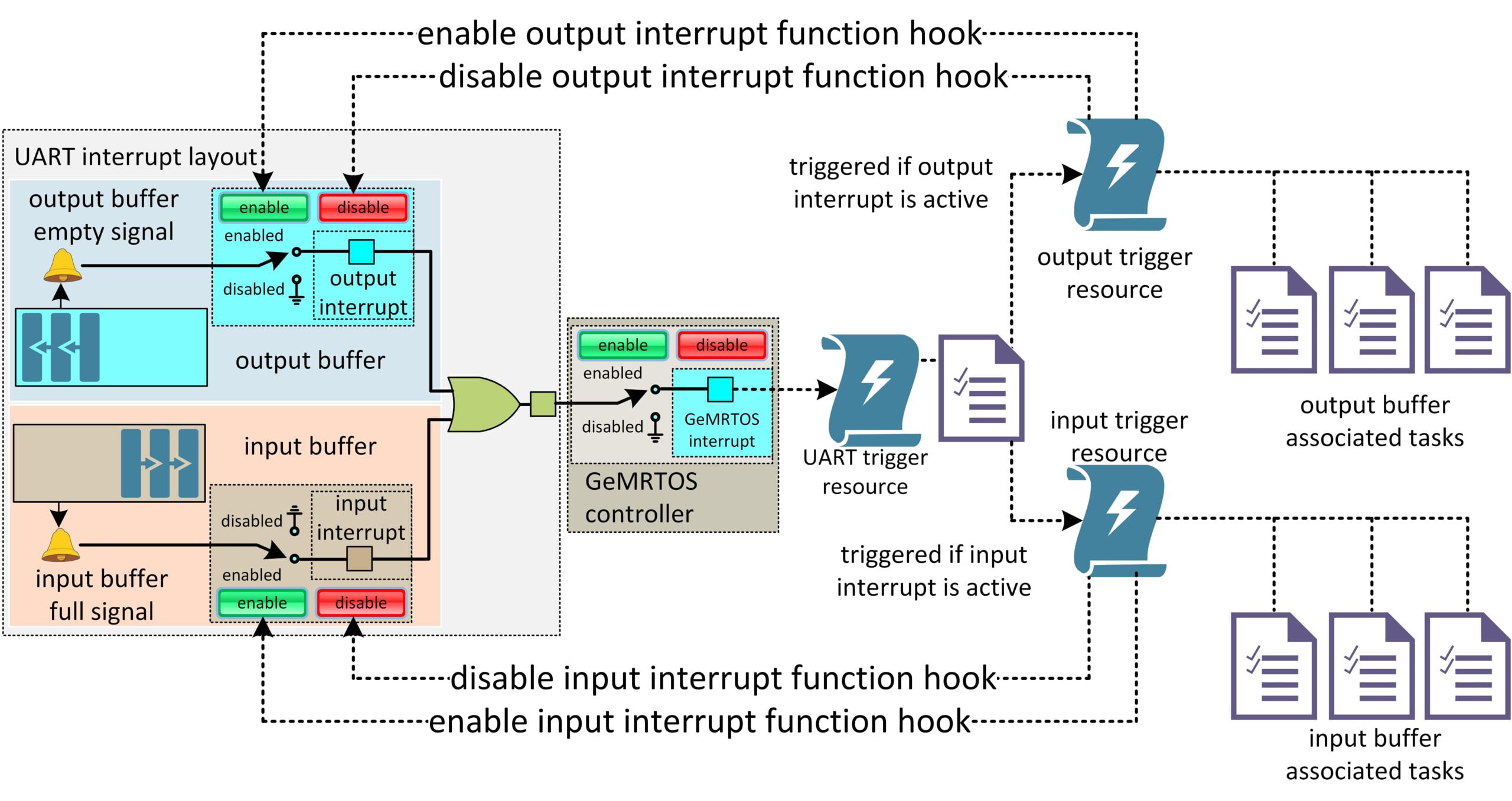
If the output buffer is empty, the system activates a trigger resource associated with writing tasks. This allows for an arbitrary number of reading and writing tasks to operate concurrently without interfering with each other. The trigger resource associated with the input buffer should have defined hook functions to enable and disable the input buffer full event. Similarly, the trigger resource associated with the output buffer should have defined hook functions to enable and disable the output buffer empty event. This approach allows for the virtualization and generalization of both internal and external events through the use of trigger resources.
Trigger related functions #
Creating and initializing a Trigger #
The first step is to create a trigger resource using:
G_RCB *gu_TriggerCreate(int IRQ_ID);
The function creates a trigger resource. It accepts an IRQ_ID argument to allow associating the trigger resource with a hardware interrupt.The trigger is created and initilized with the following paramenters: IRQ_ID: The IRQ_ID argument specifies the number of the hardware interrupt to associate with the trigger resource. Setting this argument to -1 indicates that no association with a hardware interrupt is desired.
The gu_TriggerCreate function returns a pointer to the newly created trigger resource. This pointer must be used to reference the trigger resource in all subsequent trigger-related functions.Registering a task with a trigger resource #
A task is register to a trigger resource with the following function:
G_INT32 gu_TriggerRegisterTask(struct gs_tcb *ptcb, G_INT32 irq_nbr);
The gu_TriggerRegisterTask function associates a task with a trigger resource.The task registration with the trigger resource is performed using the following parameters: ptcb: This is a pointer to the GS_TCB structure of the task to be associated with the trigger resource.
irq_nbr: This is either the IRQ number of the hardware interrupt associated with the trigger or the pointer to the trigger resource returned by the gu_TriggerCreate function, cast to an integer (int).
The gu_TriggerRegisterTask function returns G_TRUE if the task registration with the trigger resource is successful; otherwise, it returns G_FALSE.Enabling and disabling a trigger resource #
Enabling a trigger resource #
A trigger resource can be enabled with the following function:
G_INT32 gu_TriggerEnable(int IRQ_ID);
The gu_TriggerEnable function enables the trigger resource, allowing it to be activated using either the gu_TriggerRelease function or the associated hardware interrupt.The enabling of the trigger resource is performed with the following parameters: IRQ_ID: This parameter represents either the IRQ number of the hardware interrupt associated with the trigger resource or the pointer to the trigger resource returned by the gu_TriggerCreate function. If the IRQ_ID is a pointer, it should be cast to an integer (int) for use within the function.
The function returns G_TRUE if the operation was successful.Disabling a trigger resource #
A trigger resource can be disabled with the following function:
G_INT32 gu_TriggerDisable(unsigned int IRQ_ID);
The gu_TriggerDisable function disables the trigger resource, preventing it from being activated using either the gu_TriggerRelease function or the associated hardware interrupt.The disabling of the trigger resource is performed with the following parameter: IRQ_ID: This parameter represents either the IRQ number of the hardware interrupt associated with the trigger resource or the pointer to the trigger resource returned by the gu_TriggerCreate function. If the IRQ_ID is a pointer, it should be cast to an integer (int) for use within the function.
The function returns G_TRUE if the operation was successful.Waiting for a trigger resource #
A task registered to a trigger resource can wait for a triggering with the following function:
G_INT32 gu_TriggerWait(void);
The gu_TriggerWait function places the task into a waiting state for the trigger resource it is registered to. It can be executed anywhere in the task's code, and the same effect occurs when the task completes its execution (assuming it's not an infinite loop).The gu_TriggerWait function does not require any parameters, as the task automatically waits for the trigger resource it is associated with.The function returns G_TRUE if it is executed from within a task's code; otherwise, it returns G_FALSE if it is executed from the main code.Releasing a trigger resource #
A trigger resource may be triggered with the following function:
G_INT32 gu_TriggerRelease(int irq_nbr);
The function activates a trigger resource. If the trigger resource is enabled and all associated tasks are in a waiting state for the trigger, then the tasks are resumed or restarted.The trigger resource is activated using the following parameter: irq_nbr: This parameter represents either the IRQ number of the hardware interrupt associated with the trigger resource or the pointer to the trigger resource returned by the gu_TriggerCreate function. If the irq_nbr is a pointer, it should be cast to an integer (int) for use within the function.
The function returns G_TRUE if the trigger resource was successfully activated; otherwise, it returns G_FALSE.Enable and disable trigger resource hook functions #
Enable hook function #
The enable hook function specification is performed with the following function:
G_INT32 gu_TriggerEnableHook(int IRQ_ID, void *code_callback, void *p_arg);
The gu_TriggerEnableHook sets the hook function to be called before the trigger resource is enabled.The gu_TriggerEnableHook function requires the following paramenters: IRQ_ID: This parameter represents either the IRQ number of the hardware interrupt associated with the trigger resource or the pointer to the trigger resource returned by the gu_TriggerCreate function. If the IRQ_ID is a pointer, it should be cast to an integer (int) for use within the function.
code_callback: This parameter defines the name of the function to be executed as a hook function when the trigger resource is enabled.
p_arg: This parameter represents the value to be passed to the hook function when it is called. This allows the same hook function to be used for multiple trigger resources with different parameter values.
The function returns G_TRUE if the enable hook function was successfully configured; otherwise, it returns G_FALSE.Disable hook function #
The disable hook function specification is performed with the following function:
G_INT32 gu_TriggerDisableHook(int IRQ_ID, void *code_callback, void *p_arg);
The gu_TriggerDisableHook function sets the hook function to be called after the trigger resource is disabled.The disable hook function is specified using the following parameters: IRQ_ID: This parameter represents either the IRQ number of the hardware interrupt associated with the trigger resource or the pointer to the trigger resource returned by the gu_TriggerCreate function. If the IRQ_ID is a pointer, it should be cast to an integer (int) for use within the function.
code_callback: This parameter defines the name of the function to be executed as a hook function when the trigger resource is disabled.
p_arg: This parameter represents the value to be passed to the hook function when it is called. This allows the same hook function to be used for multiple trigger resources with different parameter values.
The function returns G_TRUE if the disable hook function was successfully configured; otherwise, it returns G_FALSE.Trigger resource related structure #
T_TRIGGER_RESOURCE #
The T_TRIGGER_RESOURCE is defined as the field "trigger" in a G_RCB resource. So, the fields of the T_TRIGGER_RESOURCE structure should be addressed as: (G_RCB *)->trigger.<field>